An Introduction to CoffeeScript
Imagine a desperate situation: you are in the land of Braughsir (adjacent to the kingdoms of Marrcup and Knoad), and in order to rescue a beautiful royalty of your preferred gender you must walk a hundred miles.
Every few steps you have to spin around. And there are mines. Dropped-semicolon mines, unclosed-brace mines, global variable mines, all magical mines waiting to silently explode and blow off your leg 10 minutes after you trip over them.
Now imagine a fairy comes to help you. It promises to halve the distance, enclose the mines in walls, and give you rocket skates. Rocket skates! This fairy is CoffeeScript. You will accept its offer. However, now that you’re convinced, you’ll need rational reasons that you can tell your boss. No worries, we have those!
Reason 1: It writes better JavaScript than you do.
The first thing you should know about CoffeeScript is that it’s just JavaScript with a shiny layer of paint. Usually you write your CoffeeScript then feed it to a compiler, which spits out JavaScript. In this article, we’ll rip off that layer of paint manually, to show the plain old JavaScript underneath.
Let’s take our first example:
x = 5
If you’re coming from a language like Ruby or Python, you’ll think nothing of this. If you’re coming from JavaScript, you’ll be a little nervous. Why? Well, here’s what that statement looks like in well-written JavaScript.
var x;
x = 5;
If you forget either the var or the semicolon, JavaScript will sometimes, but not always, stop working, sometimes in another seemingly unrelated part of the code. So unlike statically compiled languages like Java, beginners and experts alike are allowed to make stupid syntax mistakes, and unlike other dynamic languages like Ruby and Python, it’s extremely easy to make these mistakes and they often fail silently.
CoffeeScript shares the clarity of the dynamic languages, while also being able to catch your most egregious errors at compile-time, like a static language. And gotchas like missing vars and dropped semicolons? Automatically handled for you. The JavaScript code that it builds for you even passes JavaScriptLint (a style guide) without warnings.
Even if you could write code that perfect, why would you want to waste those brain cycles? If CoffeeScript did nothing else besides generate clean and fast JavaScript, it would be worth it so that I could concentrate on more important things than remembering to type out semicolons. Fortunately, CoffeeScript can do a lot more.
Reason 2: It’s JavaScript, but with less characters.
We’ll keep on going with the simple changes because those are the easiest to notice, and we don’t want you distracted with them later when we go over the more important elements. Here’s a simple example function taken from the CoffeeScript website.
square = (x) -> x * x
What’s happening here? We’re declaring a function called square
, which is equal to the expression (x) -> x * x
. What is this expression doing? The important part here is the ->
. Everything to the left is variables we’re feeding into the function, and everything to the right is what we’re doing with those variables. Here we suck in a variable x
, and multiply it by itself. Then we call it square
as a shorthand, so that we can just call square
whenever we want to multiply something by itself. square(4)
turns into 4 * 4, which turns into 16. JavaScript does the exact same operation, but it takes a lot longer to do it:
var square;
square = function(x) {
return x * x;
};
Notice that nothing that was added in the JavaScript version has anything to do with squaring numbers. It’s all just noise. CoffeeScript is the noise-blocking headphones of programming languages. However, sometimes the curly braces aren’t just noise. After all, sometimes you want to write a multi-line function. How will you keep track of where it ends without curly braces? Check out another example taken from the CoffeeScript home page:
if happy and knowsIt
clapsHands()
chaChaCha()
else
showIt()
You’ll notice that even though there are multiple lines, CoffeeScript still doesn’t have curly braces. It gets away with that because it is whitespace delimited. If you’re familiar with Python, Haml, or Sass, then you already know what this means. Basically, instead of using curly braces to control the flow, it looks at how many spaces and tabs are at the beginning of the line. Going in one more tab is basically like putting an opening curly brace, and going back one tab is like putting a closing curly brace. If this sounds complicated, don’t worry; 95% of the time, this is how well-styled JavaScript is spaced anyway.
Everything we’ve talked about so far has been basic JavaScript with a lot of tiny impediments removed. This is, indeed, one of the biggest and easiest to realize value propositions provided by CoffeeScript, but there is so much more, starting with classes.
Reason 3: It’s JavaScript, with a traditional class system
Every object-oriented language’s class system has quirks. JavaScript’s classes aren’t even called classes. They’re called “prototypes”. And yes, if you come from any other language, you will be confused, at least temporarily. Good news! CoffeeScript fixes that. Here’s a quick class and superclass arrangement:
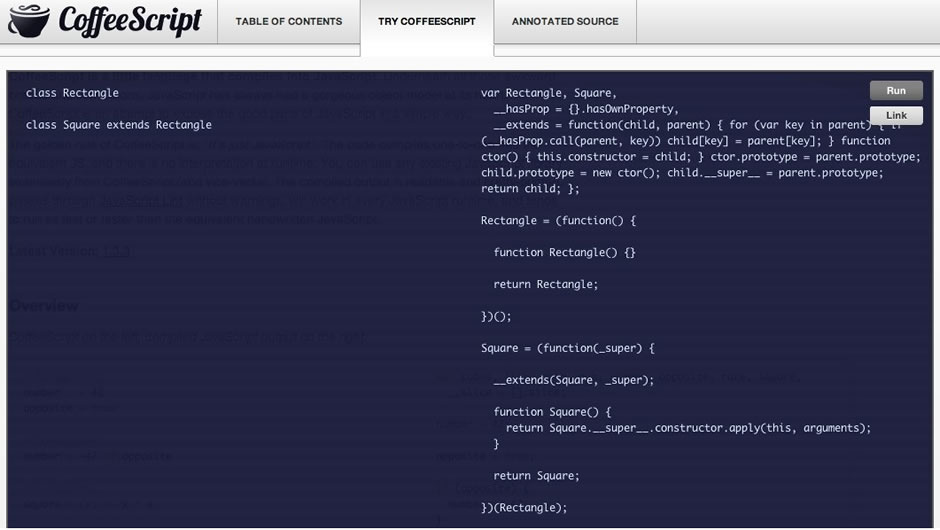
No longer do we have a line-for-line translation of CoffeeScript to JavaScript. This is because there’s a lot of contortions that must be done to fit a traditional class system into JavaScript’s prototype system. Without CoffeeScript, your mind would have to perform these contortions on its own. Here’s a basic but more complete example with CoffeeScript classes, so you can start using them right away:
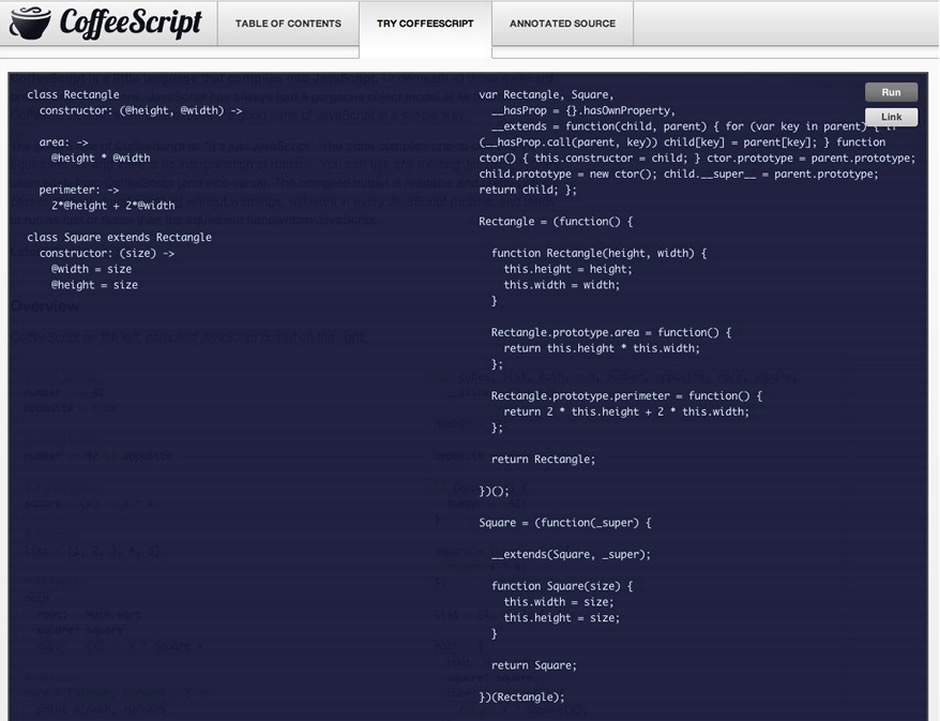
CoffeeScript in the Wild
Lots of the examples I’ve used have been taken from the CoffeeScript homepage. They have a nice translator there, which will take your CoffeeScript and immediately show you what the corresponding JavaScript will look like.
However, if you’re going to use it in real life, you’re going to need a little something more. If you’re using Ruby on Rails, rename your .js
files to either .coffee
or .js.coffee
. Now you’re done. It will even minify and concatenate your JavaScript files for you. If you aren’t using Ruby on Rails, then you’re going to have to download and install node.js and its corresponding CoffeeScript package. Then set it to watch your CoffeeScript files.