The new Cartamundi Nordic website has a clear focus on what our Swedish local office can offer to the customers in Scandinavia and tells you lot’s of stories on how we’ve created card games and board games for Scandinavian customers in the past.
Stories and Cases
Cartamundi makes cards and games, since 1765. But what exactly is it that we do? Check out the new cases page. This page will be the home of all products – in all categories. Looking for board games, or trading cards? It’s all there, with lot’s more to follow.
At present the content is limited. New cases will be added on a regular basis, all nicely illustrated and with the complete back story. Do you want your case featured on the website? Just let us know. All cases can be easily shared through social media. Just click on one of the share buttons at the bottom of the page.
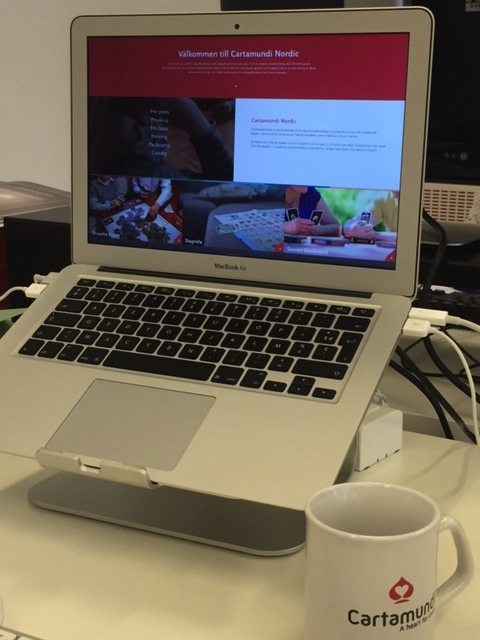
Lots to discover
Just stroll around to discover the rich content on the new Cartamundi Nordic site.We would really like to learn what you think about our new web presence. Just leave a message to our Nordic team!
Discover now on cartamundi.se!
Published on
Share this release